关于本站
“最难不过坚持”
本人承接扒站仿站,php网站维护,病毒查杀,网站编辑,网站改版,html制作
有需要网站维护,改版,病毒查杀,网站编辑,网站备案,html制作等相关的工作可以联系我。
本人有多年相关工作经验,也可提供免费咨询,交个朋友。
有需要探讨问题的朋友,也可以加我微信,共同探讨!
微信:15011482830 QQ:408917339
2699
39
分类目录
最新评论
- https://jueru.net/
-
- :weixiao:
-
- :shuijiao: :weiqu: :zhenbang: :leng:
-
- :yiwen: :yiwen: :yiwen: :yiwen:
-
- 这个业务逻辑多少都有点奇怪了,阅读浏览次数增值在新闻详情页的控制器方法里setInc,这怎么还写进模型事件里了。如果非要用onAfterRead也可以,把新闻文章的内容单独分出来一个news_content表,然后把它和news做关联,然后给news_content表的onAfterRead事件做增值处理,这样点进新闻页内查询到文章内容时才会触发它。
-
文章标签更多
打开边栏(ESC)
关闭边栏(ESC)
1.创建处理数组的类ArrayList.php
<?php /** * ArrayList实现类 * @author liu21st <liu21st@gmail.com> */ class ArrayList implements \IteratorAggregate { /** * 集合元素 * @var array * @access protected */ protected $elements = []; /** * 架构函数 * @access public * @param string $elements 初始化数组元素 */ public function __construct($elements = []) { if (!empty($elements)) { $this->elements = $elements; } } /** * 若要获得迭代因子,通过getIterator方法实现 * @access public * @return ArrayObject */ public function getIterator() { return new \ArrayObject($this->elements); } /** * 增加元素 * @access public * @param mixed $element 要添加的元素 * @return boolean */ public function add($element) { return (array_push($this->elements, $element)) ? true : false; } // 在数组开头插入一个单元 public function unshift($element) { return (array_unshift($this->elements, $element)) ? true : false; } // 将数组最后一个单元弹出(出栈) public function pop() { return array_pop($this->elements); } /** * 增加元素列表 * @access public * @param ArrayList $list 元素列表 * @return boolean */ public function addAll($list) { $before = $this->size(); foreach ($list as $element) { $this->add($element); } $after = $this->size(); return ($before < $after); } /** * 清除所有元素 * @access public */ public function clear() { $this->elements = []; } /** * 是否包含某个元素 * @access public * @param mixed $element 查找元素 * @return string */ public function contains($element) { return (array_search($element, $this->elements) !== false); } /** * 根据索引取得元素 * @access public * @param integer $index 索引 * @return mixed */ public function get($index) { return $this->elements[$index]; } /** * 查找匹配元素,并返回第一个元素所在位置 * 注意 可能存在0的索引位置 因此要用===False来判断查找失败 * @access public * @param mixed $element 查找元素 * @return integer */ public function indexOf($element) { return array_search($element, $this->elements); } /** * 判断元素是否为空 * @access public * @return boolean */ public function isEmpty() { return empty($this->elements); } /** * 最后一个匹配的元素位置 * @access public * @param mixed $element 查找元素 * @return integer */ public function lastIndexOf($element) { for ($i = (count($this->elements) - 1); $i > 0; $i--) { if ($this->get($i) == $element) { return $i; } } } public function toJson() { return json_encode($this->elements); } /** * 根据索引移除元素 * 返回被移除的元素 * @access public * @param integer $index 索引 * @return mixed */ public function remove($index) { $element = $this->get($index); if (!is_null($element)) { array_splice($this->elements, $index, 1); } return $element; } /** * 移出一定范围的数组列表 * @access public * @param integer $offset 开始移除位置 * @param integer $length 移除长度 */ public function removeRange($offset, $length) { array_splice($this->elements, $offset, $length); } /** * 移出重复的值 * @access public */ public function unique() { $this->elements = array_unique($this->elements); } /** * 取出一定范围的数组列表 * @access public * @param integer $offset 开始位置 * @param integer $length 长度 */ public function range($offset, $length = null) { return array_slice($this->elements, $offset, $length); } /** * 设置列表元素 * 返回修改之前的值 * @access public * @param integer $index 索引 * @param mixed $element 元素 * @return mixed */ public function set($index, $element) { $previous = $this->get($index); $this->elements[$index] = $element; return $previous; } /** * 获取列表长度 * @access public * @return integer */ public function size() { return count($this->elements); } /** * 转换成数组 * @access public * @return array */ public function toArray() { return $this->elements; } // 列表排序 public function ksort() { ksort($this->elements); } // 列表排序 public function asort() { asort($this->elements); } // 逆向排序 public function rsort() { rsort($this->elements); } // 自然排序 public function natsort() { natsort($this->elements); } }2.将ArrayList.php放到项目根目录下的extend文件夹下
3.在测试文件中写入测试代码
用到的代码
$list = ['aaa','bbb','ccc','ddd']; $arraylist = new ArrayList($list); $arraylist->add('cccc'); $arraylist->unique(); echo $arraylist->toJson();
4.浏览器打开测试页面展示
赏
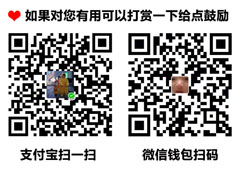
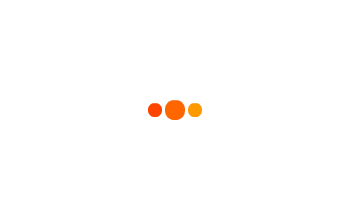
相关推荐
Non-static method think\Config::get() should not be called statically
原来是这样use think\Config;
改成这样use think\facade\Config;
下面是官方手册的解释
配置获取
要使用Config类,首先需要在你的类文件中引入
use think\facade\Config;
或者(因为系统做了类库别名,其实就是调用think\facade\Config)
u...
thinkPHP5 order多条件排序
总结如下:
//单一条件排序
$user = $this->where(['parentId'=>0)->field('userId,userName,userSort,isShow')->order('userSort', 'asc')->select();
//多个条件排序,可以多加一个order...
评论加载中...
前一篇: 5.1模板输出替换
后一篇: 怎么用composer安装第三方类库